Remember the stress of those AP Computer Science exams? Those four hours felt like an eternity as you wrestled with code and algorithms, hoping you’d be able to showcase your skills. For many students, the free-response questions (FRQs) were the most daunting part, requiring not only coding prowess but also clear communication and problem-solving abilities. If you’re looking back on your 2017 AP Computer Science experience, or if you’re a future test-taker curious about the format, this article dives into the answers to the 2017 free-response questions, offering insights into the exam’s structure and its expectations.
Image: www.chegg.com
The AP Computer Science exam, with its focus on Java, is a challenging but rewarding experience. Passing this exam can earn you college credit, making it a valuable stepping stone for students pursuing computer science degrees or careers. The free-response section plays a crucial role in the exam, accounting for a significant portion of the score. Understanding the intricacies of these questions and the strategies involved in answering them can be the key to achieving a high score.
Exam Format: A Glimpse into the Structure
The 2017 AP Computer Science exam, much like its successors, comprised two sections: a multiple-choice section and a free-response section. The free-response section, the focus of our exploration, contained four questions, each carrying a significant weight in the overall score. These questions were designed to assess the student’s ability to:
- Implement algorithms: Creating code that solves specific problems using fundamental programming concepts and data structures.
- Understand and analyze code: Interpreting given code snippets and explaining their functionalities, including potential errors or inefficiencies.
- Design and implement data structures: Building and manipulating data structures like arrays, lists, or trees to solve complex problems effectively.
- Apply principles of object-oriented programming: Utilizing concepts like inheritance, polymorphism, and data encapsulation to write modular and reusable code.
For each question, students were provided with a specific problem scenario and instructions on the desired output. The questions demanded careful analysis, logical reasoning, and efficient coding. The challenge lay in translating abstract algorithmic concepts into concrete Java code.
Question 1: The Classic Array Problem
Question 1 typically involved manipulating arrays or lists, exploring concepts like sorting, searching, or data manipulation. In 2017, students faced a problem dealing with the “mode” of an array: the most frequent element. This question tested their ability to use loops effectively to iterate through the array, count the occurrences of each element, and ultimately determine the element with the highest frequency.
Example Solution:
public static int mode(int[] nums)
if (nums.length == 0)
return -1; // Handle empty array case
Map counts = new HashMap<>(); // Store element counts
for (int num : nums)
counts.put(num, counts.getOrDefault(num, 0) + 1);
int maxCount = 0;
int mode = nums[0]; // Initialize mode
for (Map.Entry entry : counts.entrySet())
if (entry.getValue() > maxCount)
maxCount = entry.getValue();
mode = entry.getKey();
return mode;
This solution employs a HashMap for efficient counting, iterating through the array and updating the count for each encountered element. It then traverses the HashMap to find the element with the maximum count, which is the mode.
Question 2: The Recursion Challenge
Question 2 often revolved around recursive functions. Recursion is a powerful programming technique where a function calls itself to solve a smaller version of the same problem, eventually arriving at a base case. The 2017 exam presented a problem that required students to design a recursive function to identify common substrings within two given strings.
Example Solution:
public static boolean hasCommonSubstring(String str1, String str2)
if (str1.length() == 0
This solution utilizes nested loops to compare characters from both strings. It recursively checks for common substrings in the prefixes and suffixes of the strings if a matching character is found. The recursion continues until either a common substring is identified or the base case of empty strings is reached.
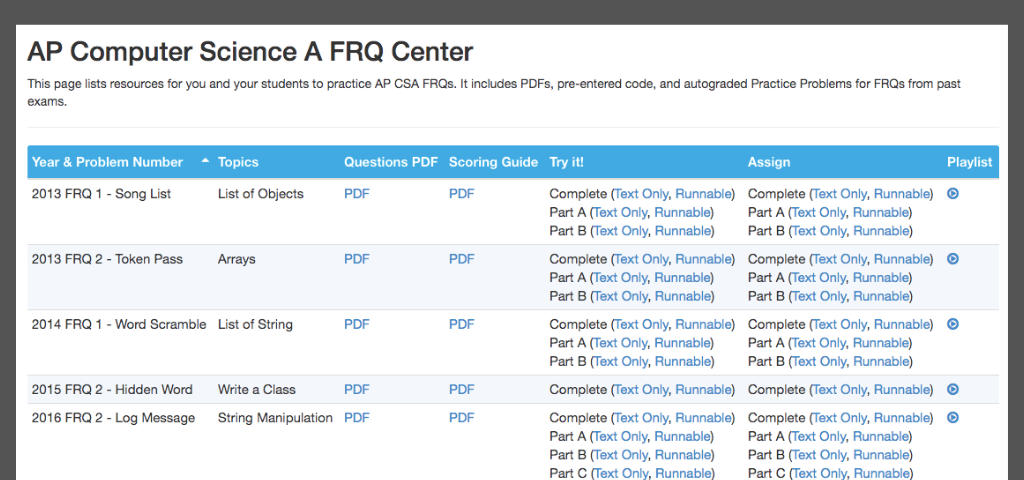
Image: reviewmotors.co
Question 3: The Object-Oriented Challenge
Question 3 typically involved object-oriented programming concepts, focusing on classes, methods, inheritance, and polymorphism. In 2017, students were presented with a scenario involving a restaurant and its menu items. They were tasked with designing and implementing classes representing dishes, categories, and a restaurant menu.
One key aspect of this question was ensuring proper inheritance and polymorphism. Methods for adding, removing, or retrieving menu items had to be implemented in a way that was efficient and reusable across different menu categories.
Question 4: The Data Structure Challenge
Question 4 generally involved the use of data structures like linked lists, stacks, queues, or trees to solve complex algorithmic problems. The 2017 exam presented a problem about a simulation of a customer queue at a store. Students had to design and implement a simulated queue using a linked list data structure, managing customer arrivals, service times, and the overall queuing process.
Tips for Success: Strategies to Master the Free-Response
Successfully navigating the free-response section requires a combination of skills and strategies:
- Practice! The more you practice, the more comfortable you’ll become with the exam’s format and the challenges posed by each question. Work through past free-response questions and engage in coding challenges to hone your problem-solving and code-writing abilities.
- Read carefully and understand the problem statement before you start coding. Identify the input, desired output, and any constraints or specific requirements.
- Plan your code before writing it. Break down the problem into smaller steps and sketch out the logic behind your solution. This helps you stay organized and avoids errors.
- Comment your code to explain your reasoning and the functionality of your code. Clear and concise comments can earn you partial credit even if your code doesn’t entirely function.
- Test your code thoroughly using different inputs and edge cases. Identify and fix any errors before submitting your solution.
- Communicate clearly in your written explanations. Use proper English and technical terms to describe your thought process and the rationale behind your code choices.
Beyond the Exam: Real-World Applications
While the AP Computer Science exam might seem like a standardized test, its concepts have profound real-world applications. Mastering these concepts equips you with the fundamental building blocks of programming, opening doors to diverse areas like:
- Software development: Creating web applications, mobile apps, and desktop software using programming languages like Java or Python.
- Data science and analytics: Analyzing large datasets to extract meaningful insights, build predictive models, and solve complex problems.
- Game development: Bringing video games to life by designing game logic, graphics, and AI.
- Cybersecurity: Defending systems from cyber threats, using programming skills to analyze vulnerabilities and build secure solutions.
2017 Ap Computer Science Free Response Answers
Conclusion: The Key to Success
The 2017 AP Computer Science free-response questions, while challenging, provide valuable insight into the types of programming problems you might encounter in your future studies or career. The key to success lies in a combination of preparation, practice, and a clear understanding of fundamental programming concepts. So, if you’re looking to advance your coding skills, master the art of algorithms, or simply gain a deeper understanding of the world of computer science, remember that the journey starts with a solid understanding of the basics – and the AP Computer Science exam can be a great stepping stone on that journey.